In this article, you’ll discover 3 ways to open a Parquet file in Python to load your data into your environment.
As a Data Scientist, it’s essential to learn how to open a Parquet file in Python.
This file format, developed by Apache, offers numerous advantages for data storage and processing.
What’s more, the open source Python libraries Pandas, PyArrow and Polars allow you to manipulate this format with ease.
You’ll find everything you need to know in this article!
What is a Parquet file?
Parquet is an open source data file format designed for efficient data storage and retrieval.
It offers significant advantages in terms of data compression and encoding, improving performance when processing large volumes of complex data.
Unlike row-based data storage formats, Parquet organizes data by columns, saving space and speeding up query processing.
Parquet is commonly used for analysis (OLAP) in conjunction with OLTP databases.
This file format has several key features.
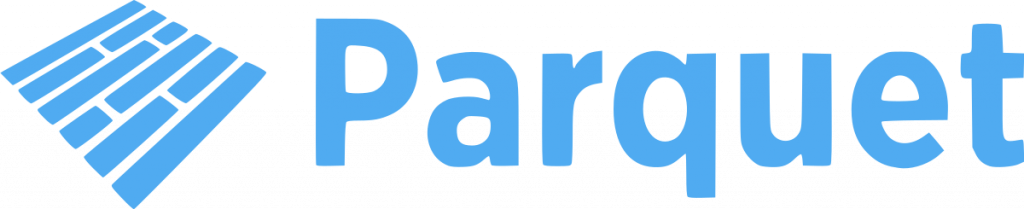
Parquet features
First of all, it’s free and open source, making it accessible to everyone.
It’s also language-independent, which means it can be used with different programming languages.
What’s more, it supports complex data types and advanced data structures. This makes it ideal for storing structured tables, images, videos and documents.
One of Parquet’s major advantages lies in its cloud storage efficiency.
Thanks to its column-based compression and encoding schemes, it saves considerable space.
In addition, it improves data throughput and performance by using techniques such as data skipping, which enables only the necessary columns to be read, thus minimizing the read load.
Parquet is the ideal solution for processing large volumes of data. It is used by the major players in the field: AWS Athena, Amazon Redshift Spectrum, Google BigQuery and Google Dataproc. It goes without saying that it is crucial to know how to process this type of file.
Open a Parquet in a Pandas DataFrame – Python
What is Pandas?
The Pandas library is the most popular tool for working with data in Python.
It offers a simple and efficient way of manipulating, analyzing and visualizing data: DataFrames. A powerful table-like data structure.
A DataFrame is a rectangular grid of rows and columns, where each column can contain data of different types such as numbers, strings or dates.
This flexibility makes DataFrames extremely versatile for handling a wide variety of data.
With Pandas, you can easily import data from CSV, Excel, SQL files – and even Parquet!
How to use it?
It’s easy to import data stored in a file in Parquet format using the Pandas library in Python.
Here’s the code to do it:
import pandas as pd
df_parquet = pd.read_parquet('/folder/file.parquet')
First, we import the Pandas library using the pd
alias. This allows us to access all Pandas functions using this shortcut.
Next, we use the read_parquet()
function to read the specified Parquet file.
This function takes as argument the path of the Parquet file we want to read.
The data extracted from the Parquet file is then stored in a DataFrame we’ve named df_parquet
.
Pandas is useful because it makes it easy to load a Parquet file into a DataFrame.
However, the data contained in your file may be massive.
In this case, Pandas may prove ineffective. But other approaches are at your fingertips!
By the way, if your goal is to master Deep Learning - I've prepared the Action plan to Master Neural networks. for you.
7 days of free advice from an Artificial Intelligence engineer to learn how to master neural networks from scratch:
- Plan your training
- Structure your projects
- Develop your Artificial Intelligence algorithms
I have based this program on scientific facts, on approaches proven by researchers, but also on my own techniques, which I have devised as I have gained experience in the field of Deep Learning.
To access it, click here :
Now we can get back to what I was talking about earlier.
Open a Parquet by Batch – Python
What is PyArrow?
The PyArrow library enables you to manipulate and process data efficiently. It also facilitates the transfer of data between different formats.
Using PyArrow, you can easily convert your data between different types (NumPy tables, Pandas DataFrames, etc.), which makes data analysis much smoother.
What’s more, this library offers exceptional performance, enabling you to manage large quantities of data quickly and efficiently.
Extract few lines from the file
PyArrow makes it possible to read large Parquet files that Pandas is unable to process.
For example, you can extract the first 350 lines of a file :
import pyarrow.parquet as pq
parquet_file = pq.ParquetFile('/folder/file.parquet')
for i in parquet_file.iter_batches(batch_size=350):
print("RecordBatch")
df_parquet = i.to_pandas()
break
We import the PyArrow library. Then open the file using the ParquetFile
class.
A loop is then run to read the contents of the file in batches of 350 items at a time. The code stops after reading the first batch (break
).
Extract the entire file
The entire file can also be read into several Dataframe
:
import pyarrow.parquet as pq
batch_dataframe = []
parquet_file = pq.ParquetFile('/folder/file.parquet')
for i in parquet_file.iter_batches(batch_size=350):
print("RecordBatch")
batch_dataframe.append(i.to_pandas())
Here, for each batch, we display RecordBatch
on the screen, then convert it into a Pandas DataFrame
which we add to the batch_dataframe
list.
Once we’ve gone through all the batches in the Parquet file, the batch_dataframe
list will contain all the data. Each item in the list represents a batch of 350 elements in the form of DataFrame
pandas.
To browse the data, we’ll need to analyze several DataFrames.
While effective, this multi-DataFrame approach can be a drawback in some projects. In particular, when the Data Scientist wishes to load all the data into a single DataFrame
.
Fortunately, there is one last option for this.
Opening a Parquet in a LazyDataFrame – Python
What is Polars?
Polars is a new library focused on the manipulation of structured data through powerful DataFrames.
These DataFrames are “lazy”, i.e. they evaluate queries only when necessary.
This considerably reduces the use of your computer’s memory and processor.
With Polars, you can load large datasets and process them quickly.
How do I use it?
Here’s how to create a LazyDataframe
from a CSV file with Polars :
import polars as pl
train = pl.scan_parquet('/folder/file.parquet')
Using the pl
alias, we import the Polars library. Next, we use the scan_parquet()
function to read the specified Parquet file in lazy mode.
As with Pandas, the data extracted from the Parquet file is then stored in a DataFrame we’ve named df_parquet
.
With Polars, it’s easy to read a large Parquet file for Data Analysis.
This is a central component of Artificial Intelligence.
Without massive data – it’s impossible to train neural networks like ChatGPT.
If you want to deepen your knowledge in the field, you can access my Action plan to Master Neural networks.
A program of 7 free courses that I’ve prepared to guide you on your journey to learn Deep Learning.
If you’re interested, click here:
sources:
- DataBricks– What is Parquet?
- Polars – User Guide
- PyArrow – Documentation
One last word, if you want to go further and learn about Deep Learning - I've prepared for you the Action plan to Master Neural networks. for you.
7 days of free advice from an Artificial Intelligence engineer to learn how to master neural networks from scratch:
- Plan your training
- Structure your projects
- Develop your Artificial Intelligence algorithms
I have based this program on scientific facts, on approaches proven by researchers, but also on my own techniques, which I have devised as I have gained experience in the field of Deep Learning.
To access it, click here :